In Android app development, the SeekBar is a commonly used UI component that allows users to select a value within a specified range by dragging a thumb along a horizontal bar. It is often used for tasks like adjusting volume, and brightness or seeking through media files.
In this tutorial, we will explore how to use SeekBar in an Android application using Kotlin programming language. We will create a simple example that demonstrates the basic functionality of a SeekBar.
Create New Project
Open Android Studio and create a new project. Give the project name SeekBarExample.
How to Create a New Project in Android Studio
To use Material SeekBar in an Android project, you need to add the Material Design dependency to your app-level build.gradle file. Go to Gradle Scripts > build.gradle(Module : app). Paste the following code in the dependencies block if not present.
implementation 'com.google.android.material:material:1.9.0'
SeekBar Layout XML
Open the activity_main.xml file from the res/layout directory. Replace the existing code with the following XML code for SeekBar.
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="SeekBar"
android:textAppearance="?attr/textAppearanceTitleLarge" />
<SeekBar
android:id="@+id/seekbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:max="100"
android:progress="22" />
</androidx.appcompat.widget.LinearLayoutCompat>
In the above layout, we have a SeekBar with an id of “seekBar” and a TextView with an id of “textView” to display the current progress value. The progress value of the SeekBar is the text size of the TextView.
SeekBar in Android Kotlin
Once you have created the layout of the SeekBar and TextView in XML, it’s time to change the size of the TextView based on the progress of the SeekBar.
Go to app > java > com.example.seekbarexample > MainActivity.kt and paste the following code.
package com.example.seekbarexample
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.SeekBar
import com.example.seekbarexample.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.seekbar.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener {
override fun onProgressChanged(p0: SeekBar?, p1: Int, p2: Boolean) {
binding.textView.textSize = p1.toFloat()
}
override fun onStartTrackingTouch(p0: SeekBar?) {
// Do nothing
}
override fun onStopTrackingTouch(p0: SeekBar?) {
// Do nothing
}
})
}
}
In the above code, we initialize the seekBar and textView variables using data binding. We also set an OnSeekBarChangeListener on the seekBar, which allows us to monitor the changes in progress and update the text size of the textView accordingly.
Testing Your App
Connect your Android device or start an emulator, then click the Run button in Android Studio to install and run the application on your device/emulator.
Once the application is launched, you will see a SeekBar with an initial progress value of 22. As you drag the thumb of the SeekBar, the progress value will change, and the text size of the textView will be updated accordingly.
Try dragging the thumb left or right to adjust the progress value and observe the changes in the TextView. Here is the final output.
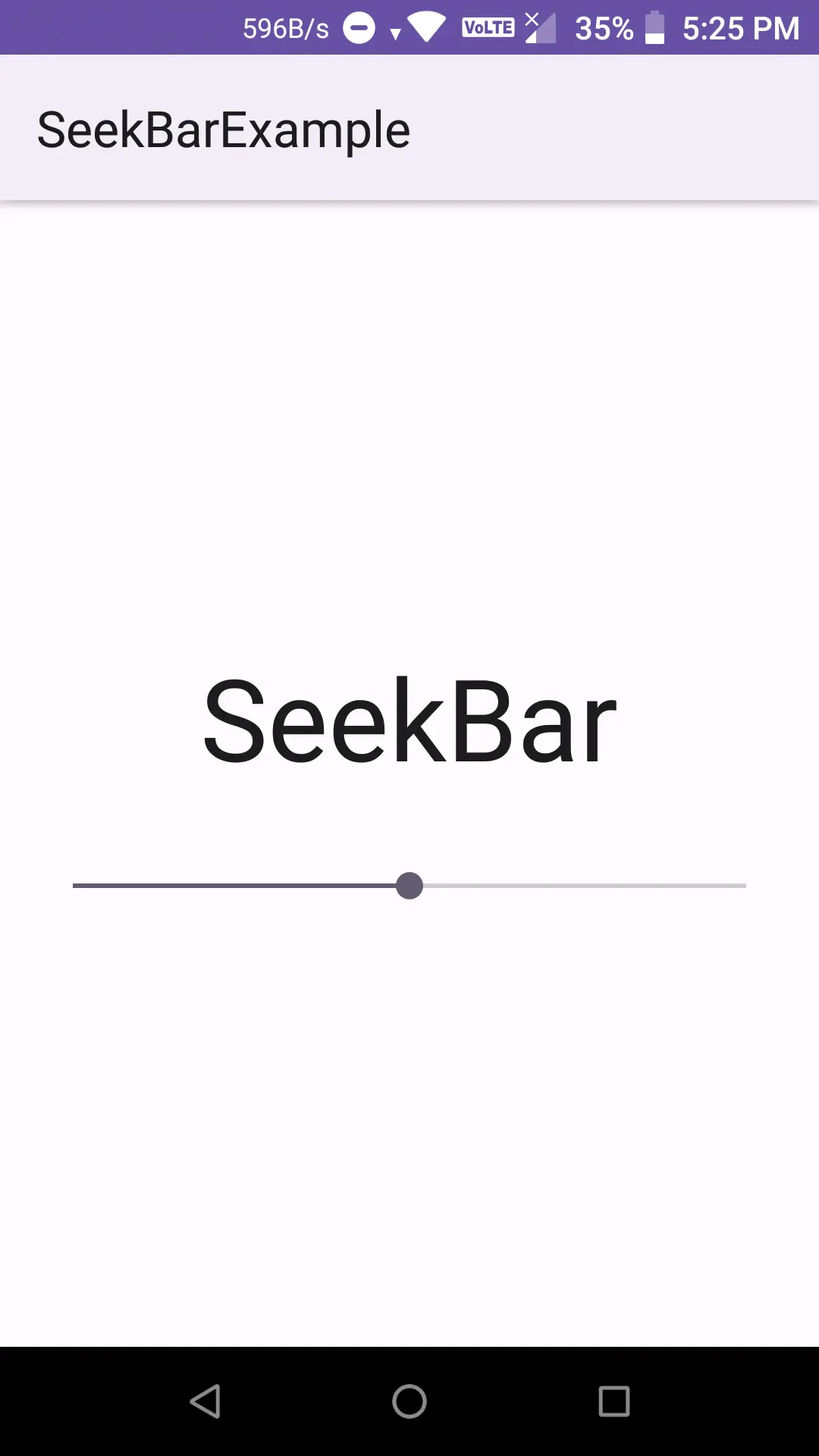
Customize the SeekBar
The SeekBar offers various attributes that you can customize to match your app’s design and functionality. Some commonly used attributes include:
- android:max: Sets the maximum value of the SeekBar.
- android:progress: Sets the initial progress value.
- android:thumb: Defines a custom drawable for the thumb of the SeekBar.
- android:progressDrawable: Defines a custom drawable for the track of the SeekBar.
Feel free to explore these attributes and experiment with different values to customize the appearance and behavior of the SeekBar.
Conclusion
In this tutorial, we learned how to use the SeekBar component in an Android application using Kotlin. We created a simple example that demonstrated how to track the progress changes of the SeekBar and update the size of TextView accordingly.
By customizing the SeekBar’s attributes, you can enhance its appearance and tailor it to your specific app requirements.
The SeekBar is a versatile UI component that provides users with an intuitive way to select values within a defined range. Incorporating it into your Android applications can greatly improve the user experience and make your app more interactive.
Feel free to expand upon this example and explore additional features and functionality offered by SeekBar to further enhance your Android applications.
So, go ahead and try it out today. Happy coding!