What is Toast in Android?
Toast messages are a crucial component in Android app development. They provide a quick and non-intrusive way to display short messages or notifications to users.
In this tutorial, we will explore how to implement toast messages in Android using Kotlin, the popular programming language for Android development.
To follow along with this tutorial, you should have a basic understanding of Android app development using Kotlin and have Android Studio installed on your machine.
Set up the Project
Open Android Studio and create a new project. Give the project name ToastMessage.
How to Create a New Project in Android Studio
Design the User Interface
Open the activity_main.xml file located in the res/layout directory. Design your user interface as per your requirements. For this tutorial, we’ll keep it simple and just use a button to trigger the toast message.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_show_toast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:minHeight="?attr/actionBarSize"
android:text="Show Toast"
android:textAppearance="?attr/textAppearanceTitleMedium"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Implementing Toast Messages
Open the MainActivity.kt file located in the app/src/main/java/com.example.toastmessage/ directory.
Inside the onCreate method, retrieve a reference to the button view by using findViewById. In this example, our button has an id of btnShowToast.
Attach an OnClickListener to the button. Inside the OnClickListener, add the code to display the toast message. Use the Toast.makeText method to create a toast message with the desired text.
package com.example.toastmessage
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.Toast
class MainActivity : AppCompatActivity() {
private lateinit var btnShowToast: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Retrieving a reference to the button view
btnShowToast = findViewById(R.id.btn_show_toast)
// Attaching an OnClickListener to the button
btnShowToast.setOnClickListener {
// Using the Toast.makeText method to create a toast message
Toast.makeText(this, "Hello, NotesJam Toast", Toast.LENGTH_LONG).show()
}
}
}
Here, this refers to the current application context, “Hello, NotesJam Toast” is the message text, and Toast.LENGTH_LONG specifies the duration of the toast message (either Toast.LENGTH_SHORT or Toast.LENGTH_LONG).
Testing Your App
Build and run the application on an emulator or physical device. Click the button, and you should see the toast message appear on the screen. Here is the final output.
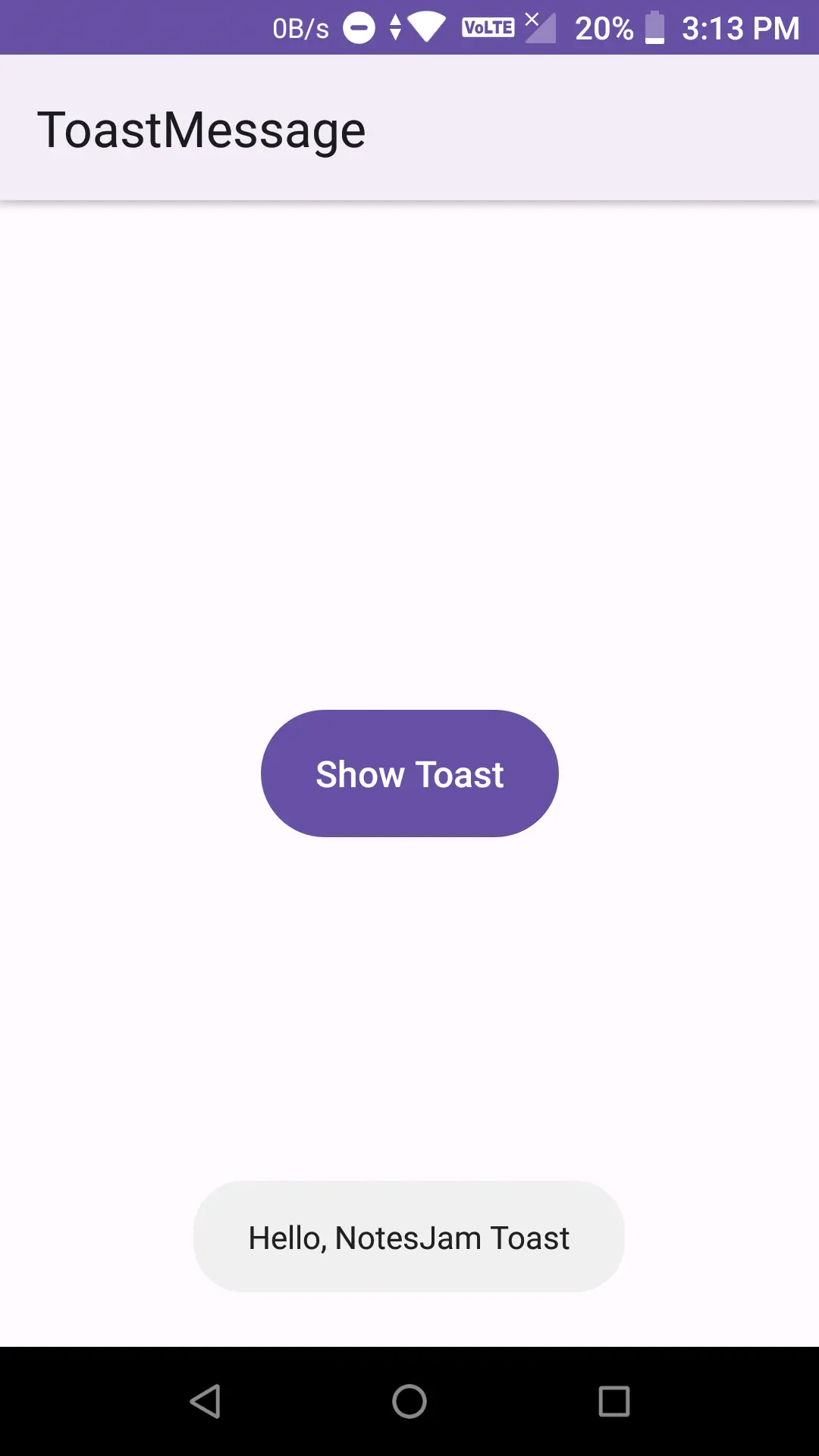
Congratulations! You have successfully implemented a toast message in your Android Kotlin application. You can customize the appearance of toast messages by changing their duration, position, and layout.
Feel free to experiment and explore the Android documentation to discover more toast message options.
Conclusion
In this tutorial, you learned how to implement toast messages in Android using Kotlin. Toast messages are an essential tool for displaying short notifications to users in a non-intrusive manner.
By following the steps outlined above, you can easily add toast messages to your Android applications. As you progress in your Android development journey, you can further enhance your toast messages by customizing their appearance and behavior.
So, go ahead and try it out today. Happy coding!